The CQGCEL encapsulates the main functionality of the CQG API.
This is the central interface an object of which should be created by the user in order to use the CQG API features.
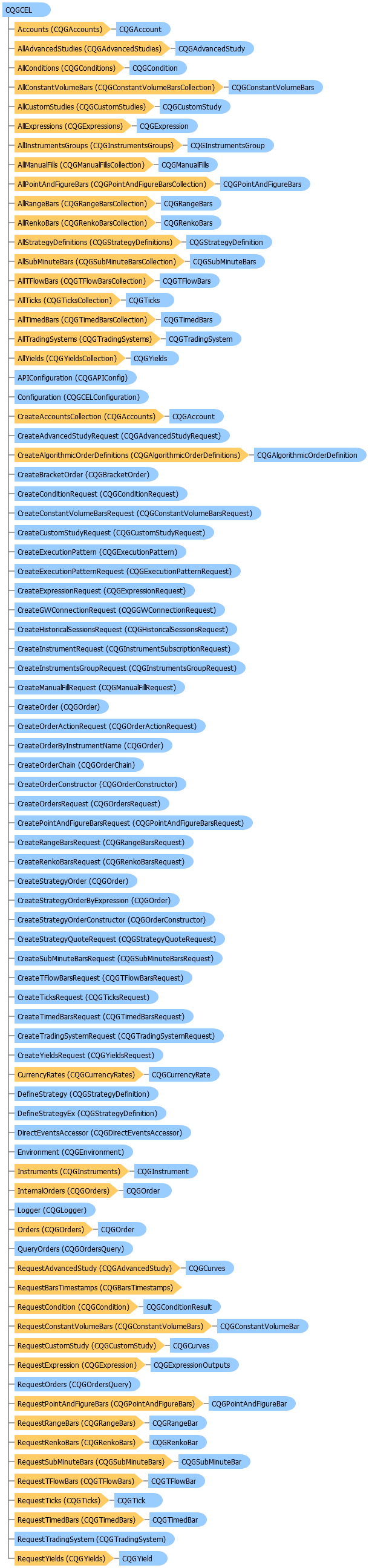
The Excel macro example below shows how to initialize/shutdown CQGCEL and handle it's events.
To execute the code, you need to add CQGCEL as reference into your project.
VB
C#
Option Explicit ' CQGCEL instance declaration Public WithEvents cel As CQGCEL Private Sub Workbook_Open() ' Initialize CQGCEL instance. On Error Goto ErrHandler Set cel = New CQGCEL cel.Startup Exit Sub ErrHandler: If Err.Number <> 0 Then MsgBox "Error occurred during CEL initialization. " & _ Err.Description End If End Sub Private Sub Workbook_BeforeClose(Cancel As Boolean) ' Shutdown the CQGCEL instance. cel.Shutdown End Sub Private Sub CEL_CELStarted() ' Create new instrument after CEL is started up cel.NewInstrument "DD" End Sub ' START of CQGCEL event handlers. Private Sub cel_InstrumentChanged(ByVal Instrument As CQG.ICQGInstrument, _ ByVal Quotes As CQG.ICQGQuotes, _ ByVal Props As CQG.ICQGInstrumentProperties) ' You'll get here if "DD" Instrument data is changed. cel.Logger.Log "Instrument Data Changed : " & Instrument.FullName, lsInfo End Sub Private Sub cel_InstrumentSubscribed(ByVal Symbl As String, ByVal Instrument As CQG.ICQGInstrument) ' You'll get here if "DD" Instrument is subscribed. cel.Logger.Log "Instrument Data Changed : " & Symbl, lsInfo End Sub Private Sub cel_IncorrectSymbol(ByVal Symbl As String) ' You'll get here if "DD" Instrument is not subscribed. cel.Logger.Log "INCORRECT SYMBOL: " & Symbl, lsError End Sub Private Sub cel_IsReady(ReadyStatus As CQG.eReadyStatus) ' CQGCEL asks Excel whether it's ready for event processing. ReadyStatus = IIf(Application.Ready, rsReady, rsNotReady) End Sub Private Sub cel_DataError(ByVal Obj As Object, ByVal ErrorDescription As String) cel.Logger.Log "ERROR : " & ErrorDescription, lsError End Sub ' END of CQGCEL event handlers.
using System ; using CQG ; using System.Runtime.InteropServices ; namespace Sample { class TestCQGCEL { // CQGCEL instance declaration. public CQGCEL cel = null ; public TestCQGCEL() { // Initialize CQGCEL instance. try { cel = new CQGCEL() ; // Attach event handlers. cel.InstrumentChanged += new _ICQGCELEvents_InstrumentChangedEventHandler( cel_InstrumentChanged ) ; cel.InstrumentSubscribed += new _ICQGCELEvents_InstrumentSubscribedEventHandler( cel_InstrumentSubscribed ) ; cel.IncorrectSymbol += new _ICQGCELEvents_IncorrectSymbolEventHandler( cel_IncorrectSymbol ) ; cel.CELStarted += new _ICQGCELEvents_CELStartedEventHandler( cel_CELStarted ) ; cel.IsReady += new _ICQGCELEvents_IsReadyEventHandler( cel_IsReady ) ; cel.DataError += new _ICQGCELEvents_DataErrorEventHandler( cel_DataError ) ; cel.Startup() ; } catch( COMException ex ) { Console.WriteLine( "Error occurred during CEL initialization. " + ex.Message ); } } ~TestCQGCEL() { // Shutdown the CQGCEL instance. cel.Shutdown() ; } #region CQGCEL event handlers private void cel_InstrumentChanged(CQGInstrument Instrument, CQGQuotes Quotes, CQGInstrumentProperties props) { // You'll get here if "DD" Instrument data is changed. cel.Logger.Log( "Instrument Data Changed : " + Instrument.FullName, eLogSeverity.lsInfo ) ; } private void cel_InstrumentSubscribed(string Symbl, CQGInstrument Instrument) { // You'll get here if "DD" Instrument is subscribed. cel.Logger.Log( "Instrument Data Changed : " + Symbl, eLogSeverity.lsInfo ) ; } private void cel_IncorrectSymbol(string Symbl) { // You'll get here if "DD" Instrument is subscribed. cel.Logger.Log( "INCORRECT SYMBOL: " + Symbl, eLogSeverity.lsError ) ; } private void cel_CELStarted() { // Create new instrument after CEL is started up cel.NewInstrument( "DD" ) ; } private void cel_IsReady(out eReadyStatus ReadyStatus) { // We are always ready to handle CQGCEL events. ReadyStatus = eReadyStatus.rsReady ; } private void cel_DataError(object Obj, string ErrorDescription) { cel.Logger.Log( "ERROR : " + ErrorDescription , eLogSeverity.lsError ) ; System.Windows.Forms.Application.Exit() ; } #endregion [STAThread] static void Main(string[] args) { TestCQGCEL test = new TestCQGCEL(); // Start Application. Will work until some error occurred. System.Windows.Forms.Application.Run() ; } } }